As a developer, you’ve likely encountered complex code snippets that left you scratching your head, wondering what each line does and how it all fits together.
Deciphering intricate code can be time-consuming and frustrating, especially when you’re working on a tight deadline. But what if you had an AI-powered assistant that could explain code to you in plain English?
In this article, we’ll explore how Claude can help you understand complex programming concepts, get started with using Claude for code explanation, and learn effective techniques for getting the most out of this powerful tool.
What Is Code Explanation?
Code explanation is the process of breaking down a piece of code and describing its functionality, purpose, and underlying logic in a way that is easy to understand. It involves translating the technical jargon and syntax into plain language, making it accessible to developers of all skill levels.
Code explanation is an essential skill for developers, as it helps them:
- Understand how a codebase works
- Identify potential bugs or inefficiencies
- Collaborate with other developers more effectively
- Onboard new team members faster
- Maintain and update code more easily
However, code explanation can be challenging, especially when dealing with complex or unfamiliar codebases.
Should Developers Use Claude for Code Explanation?
Yes! Claude is an incredibly powerful tool that can save developers countless hours of frustration and confusion. Claude can analyze code snippets and provide clear, concise explanations in simple words.
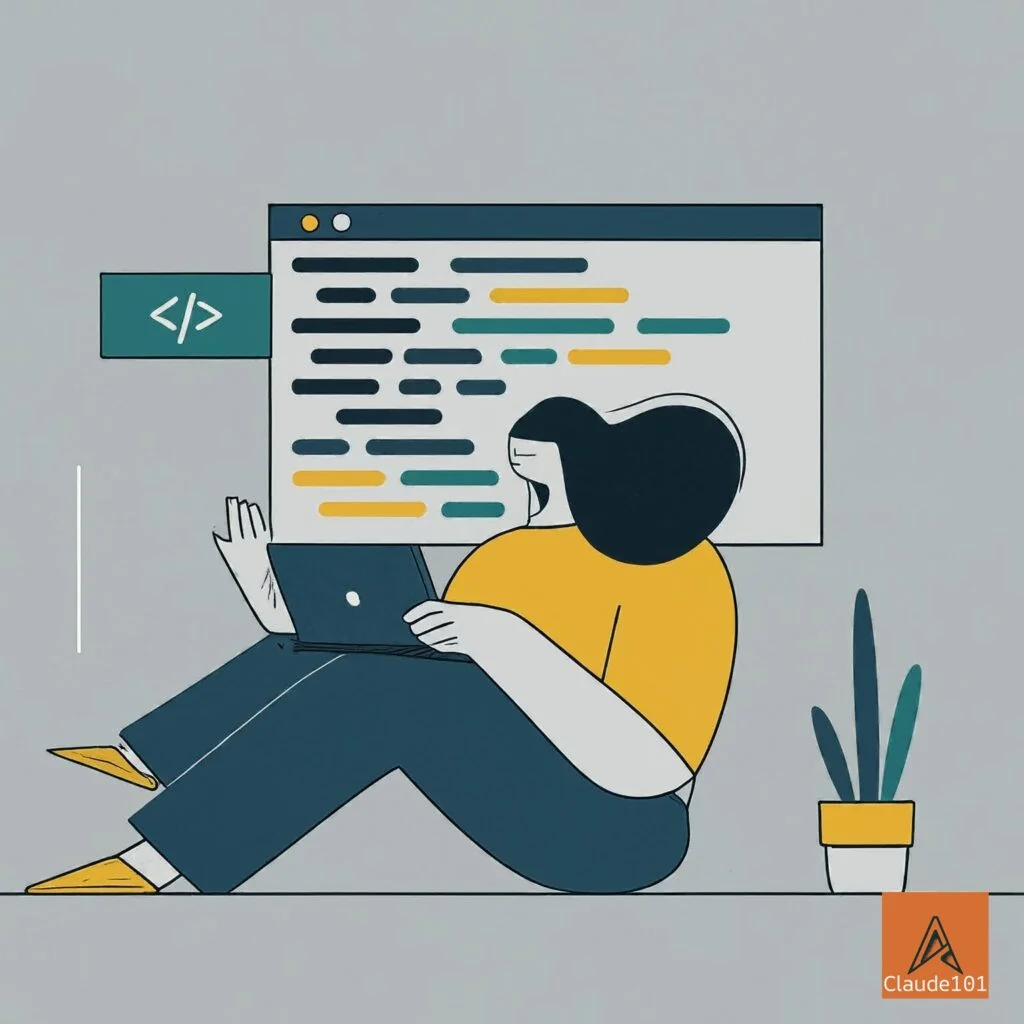
Here are just a few of the benefits of using Claude for code explanation:
- Time-saving: Claude can explain code much faster than a human developer, allowing you to quickly understand how a piece of code works without spending hours poring over documentation or debugging.
- Improved collaboration: By providing a common language for discussing code, Claude can help teams collaborate more effectively and reduce misunderstandings.
- Enhanced learning: Claude’s explanations can help developers learn new programming concepts and best practices, improving their skills over time.
- Increased productivity: With a better understanding of how code works, developers can write more efficient and effective code, leading to faster development cycles and higher-quality software.
How Can Claude Help You Understand Complex Programming Concepts?
One of the biggest challenges developers face is understanding complex programming concepts, especially when working with unfamiliar languages or frameworks. Claude can help bridge this gap by providing clear, concise explanations of even the most complex topics.
For example, let’s say you’re a Python developer who needs to work with a piece of code written in Rust. You’re not familiar with Rust’s syntax or concepts like ownership and borrowing.
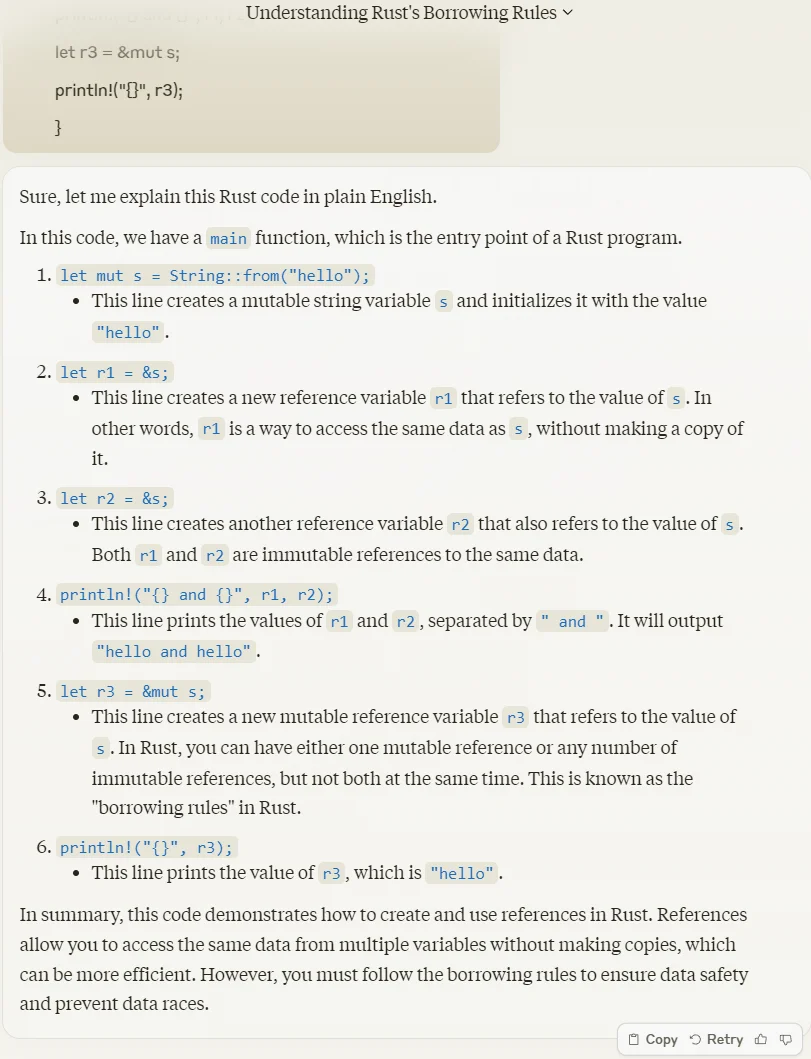
Instead of spending hours trying to decipher the code on your own, you can simply ask Claude to explain it to you:
Can you explain this Rust code to me in plain English?
fn main() {
let mut s = String::from("hello");
let r1 = &s;
let r2 = &s;
println!("{} and {}", r1, r2);
let r3 = &mut s;
println!("{}", r3);
}
Claude might respond with something like:
This Rust code defines a main function that demonstrates the concept of borrowing. Here's what each part does:
1. `let mut s = String::from("hello");` creates a mutable string variable `s` with the value "hello".
2. `let r1 = &s;` creates an immutable reference `r1` that borrows the value of `s`. This means `r1` can read the value of `s` but cannot modify it.
3. `let r2 = &s;` creates another immutable reference `r2` that also borrows the value of `s`. Multiple immutable references to the same value can exist at the same time.
4. `println!("{} and {}", r1, r2);` prints the values of `r1` and `r2`, which both point to the string "hello".
5. `let r3 = &mut s;` creates a mutable reference `r3` that borrows the value of `s`. This means `r3` can read and modify the value of `s`. However, only one mutable reference to a value can exist at a time, and no immutable references can coexist with a mutable reference.
6. `println!("{}", r3);` prints the value of `r3`, which points to the mutable string "hello".
The key takeaway is that Rust's borrowing system allows multiple immutable references or a single mutable reference to a value, but not both at the same time. This helps prevent common issues like null or dangling pointer dereferences and data races.
With Claude’s explanation, you can quickly understand how Rust’s borrowing system works and apply that knowledge to your own code.
How Do You Get Started With Claude AI for Code Explanation?
Getting started with Claude for code explanation is easy.
After logging into Claude, in the chat interface, you can start asking Claude to explain code snippets. Simply paste your code into the chat and ask Claude to explain it in plain English. For example:
Can you explain what this Python function does?
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
elif n == 2:
return [0, 1]
else:
fib = [0, 1]
for i in range(2, n):
fib.append(fib[i-1] + fib[i-2])
return fib
Claude will analyze the code and provide a clear explanation. For example:
This Python function calculates the Fibonacci sequence up to the nth number. Here's how it works:
1. If n is less than or equal to 0, the function returns an empty list since there are no Fibonacci numbers to calculate.
2. If n is 1, the function returns a list with just the first Fibonacci number, which is 0.
3. If n is 2, the function returns a list with the first two Fibonacci numbers, which are 0 and 1.
4. For any n greater than 2:
- The function initializes a list `fib` with the first two Fibonacci numbers, 0 and 1.
- It then uses a for loop to iterate from 2 to n-1. In each iteration:
- It calculates the next Fibonacci number by adding the previous two numbers in the `fib` list.
- It appends this new number to the end of the `fib` list.
- After the loop finishes, the function returns the `fib` list containing the Fibonacci sequence up to the nth number.
So if you call `fibonacci(5)`, it would return `[0, 1, 1, 2, 3]`, which are the first 5 Fibonacci numbers.
That’s it! With just a few simple steps, you can start using Claude to explain code snippets and improve your understanding of complex programming concepts.
How Do You Ask Claude to Explain Code Snippets?
Asking Claude to explain a code snippet is simple. Just paste your code into the chat interface and ask Claude to explain it in English. Here are a few tips to get the most helpful explanations:
- Be specific about what you want Claude to explain. If you have a particular question about a certain part of the code, ask about that directly.
- Provide context about the code if needed. If the code snippet is part of a larger project or relies on external libraries, let Claude know so it can give a more accurate explanation.
- Use clear and concise language in your request. Avoid ambiguous or vague questions that could lead to confusion.
Here’s an example of how you might ask Claude to explain a code snippet:
Can you help me understand what this JavaScript function does and how it works? It's part of a web scraping project I'm working on.
async function scrapeData(url) {
try {
const response = await axios.get(url);
const $ = cheerio.load(response.data);
const data = [];
$('div.product').each((i, el) => {
const name = $(el).find('h3').text();
const price = $(el).find('span.price').text();
data.push({ name, price });
});
return data;
} catch (error) {
console.error(error);
}
}
Claude would then analyze the code and provide an explanation like:
This is an asynchronous JavaScript function called `scrapeData` that scrapes product data from a webpage. Here's a breakdown of what it does:
1. The function takes a `url` parameter, which is the URL of the webpage to scrape.
2. It uses the `axios` library to send an HTTP GET request to the specified URL and waits for the response using the `await` keyword.
3. Once the response is received, it uses the `cheerio` library to load the HTML data into a parsed DOM object `$`.
4. It initializes an empty array called `data` to store the scraped product information.
5. It uses Cheerio's `each` method to loop through all the `div` elements with the class `product` on the page.
6. For each product `div`, it extracts the product name from the `h3` element and the price from the `span` element with class `price` using Cheerio's `find` and `text` methods.
7. It pushes an object containing the `name` and `price` properties to the `data` array.
8. After looping through all the products, the function returns the `data` array containing the scraped product information.
9. If any error occurs during the scraping process, it is caught in the `catch` block and logged to the console using `console.error`.
So in summary, this function scrapes a webpage for product information, extracts the product names and prices, and returns them as an array of objects. The `axios` and `cheerio` libraries simplify the process of making HTTP requests and parsing HTML.
By providing a clear and specific request, you can get a detailed explanation from Claude that helps you understand exactly how the code works.
Effective Techniques for Getting Clear Code Explanations From Claude
To get the most helpful and accurate code explanations from Claude, there are a few techniques you can use:
1. Break down complex code into smaller parts
If you have a long or complex piece of code, it can be helpful to break it down into smaller, more manageable parts before asking Claude to explain it. This way, you can get more focused explanations for each part of the code, rather than a broad overview that might miss important details.
For example, instead of asking Claude to explain an entire 100-line function, you could split it up into logical sections and ask for explanations of each section separately:
I have a long function that I'm having trouble understanding. Let's break it down into smaller parts. First, can you explain what this section of the code does?
[paste first 20 lines of code]
Then, after receiving an explanation for the first section, you can move on to the next:
Thanks, that helps clarify the first part. Now, can you explain what this next section does?
[paste next 20 lines of code]
By iterating through the code in smaller chunks, you can get more detailed and accurate explanations from Claude.
2. Provide context and ask specific questions
To get the most relevant and helpful explanations from Claude, it’s important to provide context about your code and ask specific questions. The more information you can give about what the code is supposed to do, what problem it’s trying to solve, or what specific part you’re confused about, the better Claude can tailor its explanation to your needs.
For example, instead of simply pasting a code snippet and asking for an explanation, you could say something like:
I'm working on a Python script that reads data from a CSV file and calculates some statistics. I'm having trouble understanding this part of the code that calculates the standard deviation. Can you explain how it works and what each variable represents?
[paste relevant code snippet]
By providing context about the overall script and asking a specific question about the standard deviation calculation, you’ll get a more targeted and useful explanation from Claude.
3. Iterate and ask follow-up questions
Don’t be afraid to ask follow-up questions if Claude’s initial explanation isn’t clear or if you need more information. Claude is designed to engage in multi-turn conversations, so you can keep asking for clarification or additional details until you fully understand the code.
For example, if Claude’s explanation of a particular function is too broad, you could follow up with something like:
Thanks for the explanation, but I'm still a bit confused about how the `result` variable is being calculated inside the loop. Could you go into more detail about what's happening there?
Or if Claude uses a term or concept that you’re not familiar with, you could ask for a definition or example:
You mentioned that this function uses a 'recursive' approach. Could you explain what that means and give a simple example of how it works?
By engaging in a back-and-forth dialogue with Claude, you can gradually build up your understanding of complex code snippets and programming concepts.
4. Compare Claude’s explanations to other resources
While Claude’s explanations are usually very accurate and helpful, it’s always a good idea to cross-reference them with other resources to get a well-rounded understanding of your code. You can compare Claude’s explanations to documentation, tutorials, or explanations from other developers to see if they match up and to fill in any gaps in your knowledge.
For example, after getting an explanation from Claude about a particular Python library function, you could look up that function in the official Python documentation to see if Claude’s explanation aligns with the official description and usage examples.
Or if Claude’s explanation of a complex algorithm is a bit abstract, you could search for a tutorial or blog post that walks through a concrete implementation of that algorithm to see how it works in practice.
Can I Use Claude for Other Coding Tasks?
Yes, Claude is a versatile AI that can help with a wide variety of coding and software development tasks.
Here are some of the popular ways Claude can assist programmers and developers:
Code generation
Claude can generate code snippets and even entire functions or classes based on natural language descriptions of what you want the code to do. It supports popular programming languages like Python, JavaScript, Java, C++, and more.
Code debugging Assistance
Debugging is an inevitable part of coding. When you encounter bugs or errors in your code, you can collaborate with Claude to identify and fix the issues. Share the relevant code and error messages with Claude, and it will analyze the problem and suggest solutions. Claude can pinpoint the likely source of the bug and provide corrected code.
Code refactoring and optimization
Claude can help you improve the quality, performance, and maintainability of your codebase through refactoring and optimization suggestions. Ask Claude to review a piece of code, and it will provide feedback on best practices, potential optimizations, simplifications to the logic, and adherence to standard conventions and style guides for that language.
Coding questions and advice
You can also use Claude as a knowledgeable coding assistant to ask general questions about programming languages, frameworks, libraries, algorithms, data structures, and software architecture. Claude will provide clear explanations and code examples to help you understand the concepts. It’s like having an experienced programmer available 24/7 to bounce ideas off of and get guidance from.